When you make a call to the ASP.NET Web API action method and notice this in Google Chrome browser , you would see that by default the data would be displayed in the XML format as shown in the screenshot.
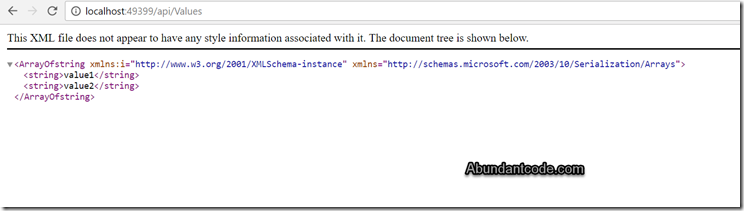
How to return Json instead of XML in ASP.NET Web API ?
You can easily change this behavior by adding the following code in the WebAPIConfig.cs file.
config.Formatters.JsonFormatter.SupportedMediaTypes .Add(new MediaTypeHeaderValue("text/html") );
This will ensure that you would get the Json on the quries unless you explicitly specify the text/xml as the Accept header.

Here’s the WebAPIConfig class file to get this to work.
using System; using System.Collections.Generic; using System.Linq; using System.Net.Http; using System.Web.Http; using Microsoft.Owin.Security.OAuth; using Newtonsoft.Json.Serialization; using System.Net.Http.Headers; namespace WebApplication1 { public static class WebApiConfig { public static void Register(HttpConfiguration config) { config.MapHttpAttributeRoutes(); config.Routes.MapHttpRoute( name: "DefaultApi", routeTemplate: "api/{controller}/{id}", defaults: new { id = RouteParameter.Optional } ); config.Formatters.JsonFormatter.SupportedMediaTypes.Add(new MediaTypeHeaderValue("text/html")); } } }
Leave a Reply