Below is a sample code snippet demonstrating the use of function delegates in C# to find the factorial of the number.
How to find the factorial of a Number using Function Delegates in C# ?
using System; using System.Collections.Generic; namespace AbundantCodeConsoleApp { internal class Program { private delegate void result(); private static void Main(string[] args) { System.Func<long, long> Fact = delegate(long Input) { if (Input == 1) return 1; long Output = 1; for (int indexer = 2; indexer <= Input; indexer++) Output *= indexer; return Output; }; Console.WriteLine(Fact(4)); Console.ReadLine(); } } }
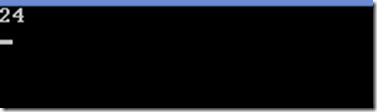
Leave a Reply