Do you want to get the distinct records from a list based on a particular property using LINQ in C#?. Below is a code snippet that demonstrates how to do it.
How to Apply LINQ Distinct on a particular property in C#?
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; namespace AbundantcodeConsole { class Program { static void Main(string[] args) { //How to App LINQ Distinct on a particular property in C# ? List emps = new List(); emps.Add(new Employee { Name = "Martin", Designation = "ACEngineer" }); emps.Add(new Employee { Name = "Mike", Designation = "ACSeniorEngineer" }); emps.Add(new Employee { Name = "Peter", Designation = "ACEngineer" }); emps.Add(new Employee { Name = "Mark", Designation = "ACEngineer" }); var employeeData = (from p in emps group p by new { p.Designation } into distinctDesignationGroup select distinctDesignationGroup.First()); foreach (var data in employeeData) Console.WriteLine(data.Name + " , " + data.Designation); Console.ReadLine(); } } public class Employee { public string Name { get; set; } public string Designation { get; set; } } }
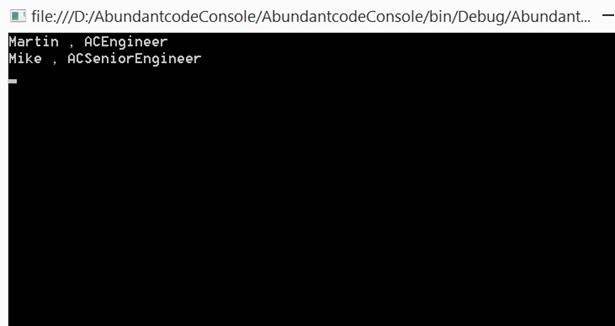
Leave a Reply