There are times when you want to get the necessary information from the exception to identify the error better in C# . The Exception class contains various properties which can be used to retreive the necessary information from the error.
Some of the properties includes
- Message – The Message property provides the brief description of error.
- Source – The Source property provides information about the application where the exception occurred.
- StackTrace – The StackTrace is one very useful property which provides information to retrace the path that caused the exception.
- InnerException – This property can be used to identify the inner exception (if any).
How to Get Necessary Information from the Exception in C# ?
Below is a sample code snippet demonstrating how to get necessary information from the exception in C#.
using System; using System.Collections.Generic; using System.ComponentModel; using System.Linq; using System.Text; namespace AbundantcodeConsole { class Program { static void Main(string[] args) { try { int i = 0, j = 0; var ret = i / j; } catch(DivideByZeroException e) { Console.WriteLine(e.Message); Console.WriteLine(e.Source); Console.WriteLine(e.StackTrace); } Console.ReadLine(); } } }
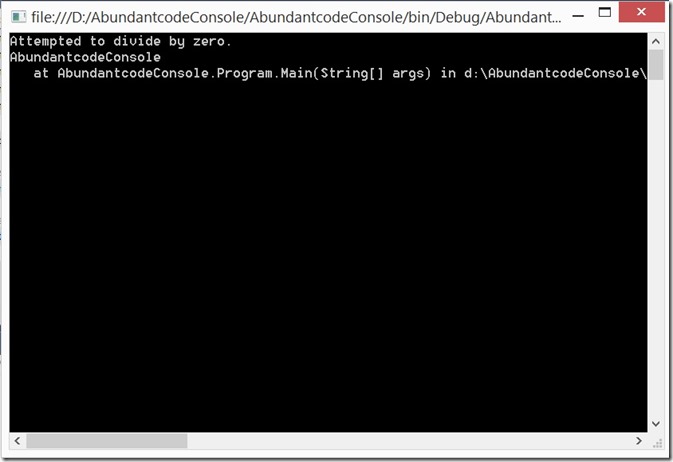
Leave a Reply