The Java’s Arrays class provides a static function called Arrays.copyOfRange that helps the developers to copy a range of elements from one array to another.
How to Copy range of elements from an array in Java ?
Below is an example that demonstrates the copying of the range of objects from one array to another using Arrays.copyOfRange.
package com.abundantcode; import java.util.Arrays; import java.util.List; public class Main { public static void main(String[] args) { String[] input = {"Java", "Abundantcode.com", "MCA Lab Programs", "B.E Java Lab Programs", "BCA Java Programs"}; System.out.println("Source Array"); System.out.println("------------"); for(String item:input) System.out.println(item); String[] outPutArray = Arrays.copyOfRange(input, 1, 3); System.out.println("Destination Array"); System.out.println("-----------------"); for(String item:outPutArray) System.out.println(item); } }
Output
Source Array
————
Java
Abundantcode.com
MCA Lab Programs
B.E Java Lab Programs
BCA Java Programs
Destination Array
—————–
Abundantcode.com
MCA Lab Programs
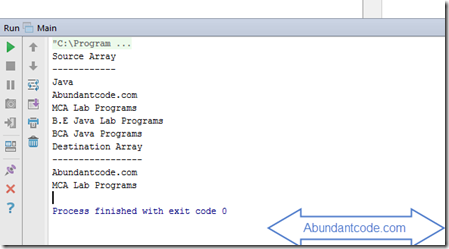
Leave a Reply