One of the ways to Deserialize an object to JSON string in C# in the Json.NET is using the DeSerializeObject method defined in the JsonConvert method.
How to Deserialize an Object in C# using Json.NET ?
Below is a sample code snippet demonstrating how you can deserialize an object from Json string to C# object using Json.NET. It takes the json string that contains the employee information and deserializes it to the Employee class.
using System; using System.Collections.Generic; using Newtonsoft.Json; namespace ACConsoleCSharp { class Program { static void Main(string[] args) { string jsonTxt = @"{'Name': 'Abundantcode', 'IsPermanent': true, 'Departments': [ 'Technology', 'Product Engineering' ] }"; Employee emp = JsonConvert.DeserializeObject<Employee>(jsonTxt); Console.WriteLine(emp.Name); Console.ReadLine(); } } public class Employee { public string Name { get; set; } public bool IsPermanent { get; set; } // Employee can belong to multiple departments public List<string> Departments { get; set; } } }
The Deserialized object contains the following data.
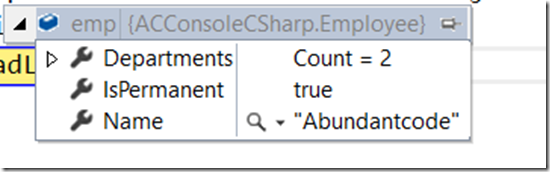
Leave a Reply