In C#, you can calculate the age based on the datetime property by subtracting the number of years from today with the birthday year. We should also consider the Leap Year as needed.
How to Calculate age based on DateTime of Birthday in C# ?
Here’s a simple code snippet demonstrating how to do it.
using System; namespace ConsoleApp5 { class Program { static void Main(string[] args) { var birthday = new DateTime(1992, 10, 02); var age = DateTime.Today.Year - birthday.Year; if (birthday.Date > DateTime.Today.AddYears(-age)) age--; Console.WriteLine("Age = " + age); Console.ReadLine(); } } }
Output
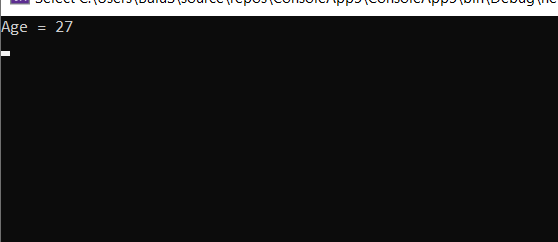
Leave a Reply