Problem
Write a program in C++ to calculate arithmetic mean for the numbers entered by the user.
Solution
We have a formula to find the arithmetic mean if there are n sets of numbers:
am = (n1+n2+n3+...+nn)/n
The letters am stand for arithmetic mean, whereas n1, n2, n3, and nn stand for first, second, third, and fourth numbers, respectively.
Here’s a sample code snippet demonstrating how you can find the arithmetic mean in C++.
#include<iostream> using namespace std; int main() { int n, i; float arr[50], sum=0, armean; cout<<"Enter the Limit"; cin>>n; cout<<"\nEnter the Number: "; for(i=0; i<n; i++) { cin>>arr[i]; sum = sum+arr[i]; } armean = sum/n; cout<<"\nArithmetic Mean = "<<armean; cout<<endl; return 0; }
Output
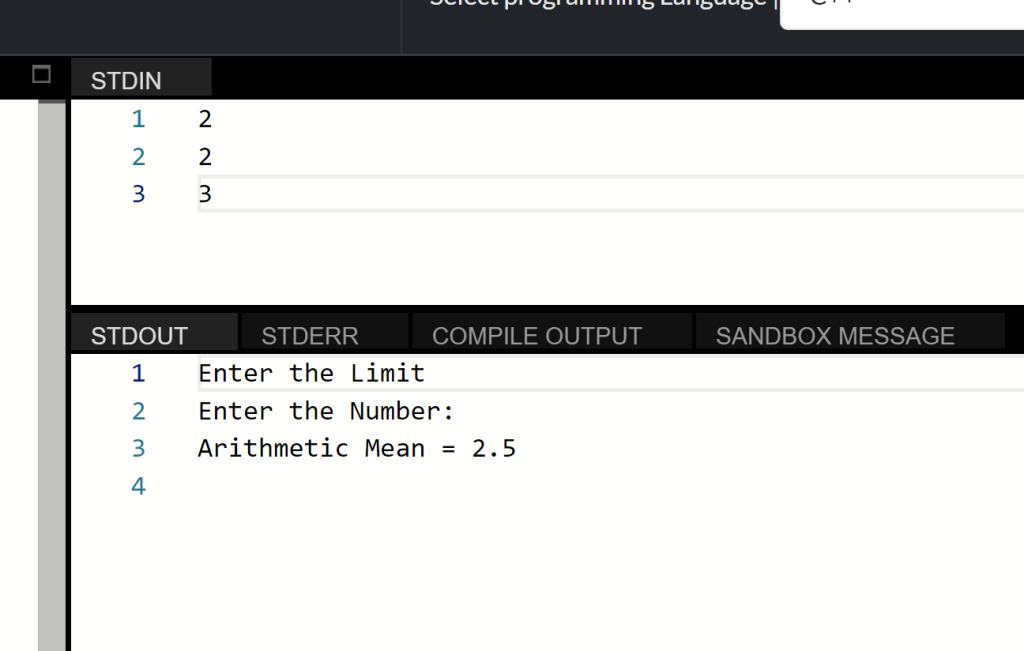
Leave a Reply