This post calculates the power using recursion by the use of C programming language.
C program to calculate the power using recursion
#include <stdio.h> int power(int n1,int n2); int main() { int base,a,result; printf("Enter base number: "); scanf("%%d",&base); printf("Enter power number: "); scanf("%%d",&a); result=power(base,a); printf("%%d^%%d = %%d",base,a,result); return 0; } int power(int base, int a) { if(a!= 0) return(base*power(base,a-1)); else return 1; }
Output
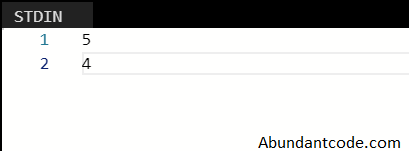
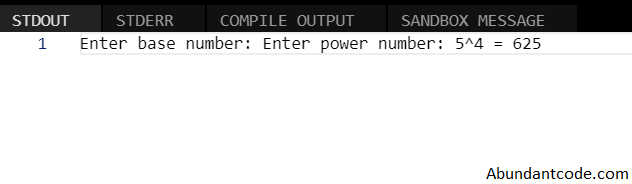
Explanation
The programs get started by initializing the functions and the variables. In this program, the variables initialized to store the values are base to get the base value, a to the get the power value and result for printing the output. We end the main function with giving return 0.
int power(int n1,int n2); int main() { int base,a,result; printf("Enter base number: "); scanf("%%d",&base); printf("Enter power number: "); scanf("%%d",&a); result=power(base,a); printf("%%d^%%d = %%d",base,a,result); return 0; }
Here we call the function to calculate the power using recursion. We also use the if condition to calculate the power, where we check the condition whether it’s true or false, and then proceed further.
int power(int base, int a) { if(a!= 0) return(base*power(base,a-1)); else return 1; }
After the if condition is satisfied, we get the output as desired.
Leave a Reply