This post helps us to display prime numbers between two intervals using C programming.
C Program to Display Prime Numbers Between Two Intervals
#include<stdio.h> int main() { int num1,num2,i,flag; printf("Enter the intervals:"); scanf("%%d %%d",&num1,&num2); printf("Prime number between %%d and %%d are:",num1,num2); //value until num1 is not equal to num2 while(num1<num2) { flag=0; //leave the numbers less than num2 if(num1<=1) { ++num1; continue; } //if num1 is non-prime number for(i=2;i<=num1/2;++i) { if(num1%%i==0) { flag=1; break; } } //for checking the prime number for the next number //increment num1 by 1 if(flag==0) printf("%%d",num1); ++num1; } return 0; }
Output
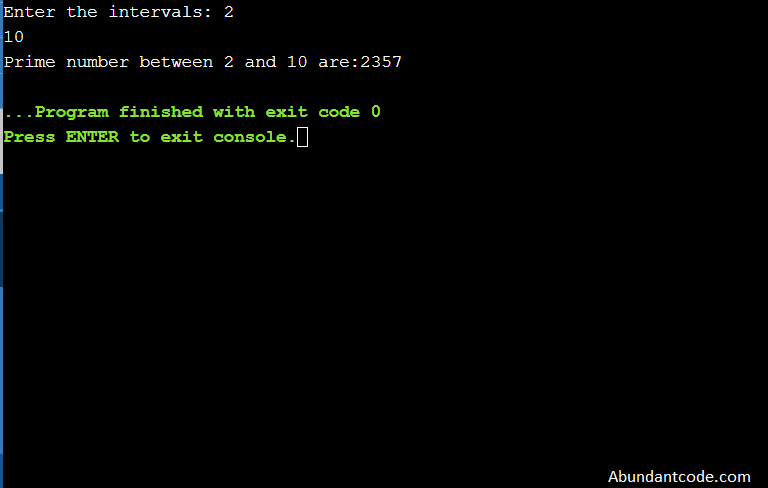
Explanation
In this C program, we find prime numbers between two intervals. Here we use the flag as a variable that acts as a signal for a function or process. A flag is often binary, which contains a boolean value(true or false).
We get the values of the intervals from the user. Now we use a while loop to check whether the given num1 value is not equal to num2 value. If the num1 is not equal to num2, then the value of the flag is declared as zero so that the prime numbers are displayed between the given intervals. If the num1 value is less than two, then it gets incremented, and the process continues until the required result is obtained.
//value until num1 is not equal to num2 while(num1<num2) { flag=0; //leave the numbers less than num2 if(num1<=1) { ++num1; continue; }
Now using for loop, we declare the value for “i” as two because one is neither or nor a prime number. The value of I gets incremented until it satisfies the “I <=num1/2” condition. Using the if statement, we make sure that the num1 value is equal to zero. If yes, then the flag will be equal to 1, and the control comes out of the loop. This if statement is to check whether the values are prime numbers or non-prime numbers.
//if num1 is non-prime number
//if num1 is non-prime number for(i=2;i<=num1/2;++i) { if(num1%%i==0) { flag=1; break; }
So we check whether the following numbers are prime numbers or not by incrementing num1 values by 1.
//for checking the prime number for the next //increment num1 by 1 if(flag==0) printf("%%d",num1); ++num1;
If the flag =0. Then the result gets displayed.
Leave a Reply