How to Convert a Byte Array to Integer in C# ?
To Convert a Byte array back to the integer , the developers can use the BitConverter.ToInt32 method which accepts byte array as input and returns the number .
Below is a sample code snippet that demonstrates how to convert a byte array to integer in C#.
How to Convert a Byte Array to Integer in C# ?
using System; using System.Collections.Generic; using System.Globalization; using System.Linq; using System.Text; namespace AbundantcodeConsoleApp { internal class Program { private static void Main(string[] args) { byte[] output = ConvertNumberToByteArray(); Int32 num = ConvertByteArrayToInteger(output); Console.WriteLine(num); Console.ReadLine(); } // Function to Convert Byte Array to Integer private static int ConvertByteArrayToInteger(byte[] output) { Int32 num = BitConverter.ToInt32(output, 0); return num; } // Function to Convert a Number to Byte Array private static byte[] ConvertNumberToByteArray() { Int32 Input = 75; byte[] ByteData = BitConverter.GetBytes(Input); return ByteData; } } }
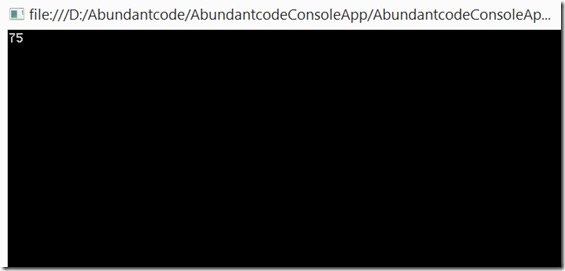
Leave Your Comment