How to find the reverse of a number using C# ?
Below is a sample code snippet that demonstrates how to find the reverse of a number using C# .
How to find the reverse of a number using C# ?
using System; using System.Collections.Generic; using System.Linq; using System.Text; namespace AbundantcodeConsoleApp { internal class Program { private static void Main(string[] args) { // How to find the reverse of a number using C# ? int Input = 2985; int ReverseNumber = 0; while (Input > 0) { int rem = Input % 10; ReverseNumber = (ReverseNumber * 10) + rem; Input = Input / 10; } Console.WriteLine("Output : {0}", ReverseNumber); Console.ReadKey(); } } }
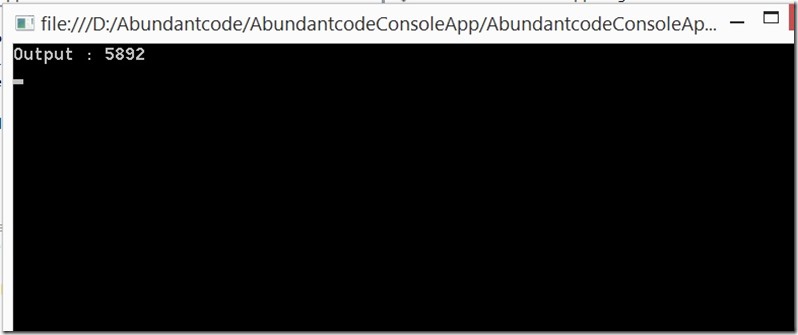
3 Comments
Or you could be a linq-fanatic and implement in a one-liner:
private static void Main(string[] args)
{
Func LinqReverse = x => x.ToString().Reverse().Aggregate(0, (a, c) => a * 10 + (c – ‘0’));
Console.WriteLine(LinqReverse(123456));
Console.ReadKey();
}
Actually: Func LinqReverse = x => x.ToString().Reverse().Aggregate(0, (a, c) => c – ‘0’ + 10 * a);
Or this:
Func LinqReverse = x => Convert.ToInt32(string.Join(string.Empty, x.ToString().Reverse()));