There are times when you have to remove duplicate entried from a List from Your C# program and one of the easiest way to do that is using the combination of the LINQ and IEqualityComparer interface.
Assume that you have a list of Actors as shown below
ID : 1 , Name = Vijay ,Language = Tamil
ID : 2 , Name = Rajni ,Language = Tamil
ID : 3 , Name = Vijay ,Language = Kannada
ID : 4 , Name = Ajith ,Language = Tamil
You want to remove the duplicates from list with the criteria that name should always be distinct. In this case, you want to remove both the items with the name = Vijay from the list.
How to Remove Duplicates in List using LINQ in C#?
Below is a sample code snippet demonstrating the usage of IEqualityCOmparer and LINQ’s Distinct method to remove one of the duplicate records based on the name.
using System.Collections.Generic; using System.Linq; using System; public class Hello { public static void Main() { List<Actor> actors = new List<Actor>(); actors.Add(new Actor { ID = 1 , Name = "Vijay" , Language = "Tamil" }); actors.Add(new Actor { ID = 2 , Name = "Rajni" , Language = "Tamil" }); actors.Add(new Actor { ID = 3 , Name = "Vijay" , Language = "Kannada" }); actors.Add(new Actor { ID = 4 , Name = "Ajith" , Language = "Tamil" }); var distinctActors = actors.Distinct(new DistinctItemComparer()); foreach(var actor in distinctActors) System.Console.WriteLine(actor.Name); } public class Actor { public int ID { get;set; } public string Name { get;set; } public string Language { get;set; } } class DistinctItemComparer : IEqualityComparer<Actor> { public bool Equals(Actor x, Actor y) { return x.Name == y.Name; } public int GetHashCode(Actor obj) { return obj.Name.GetHashCode() ; } } }
Output
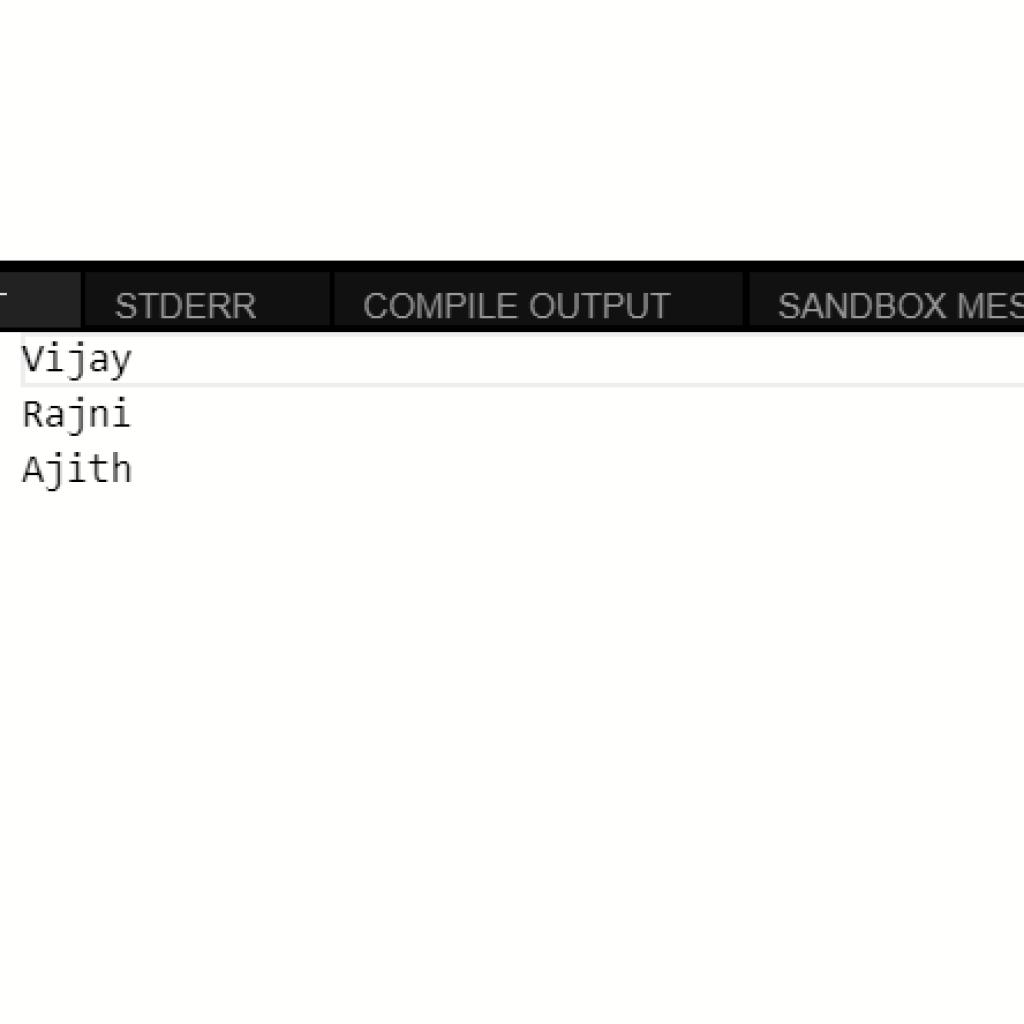
Leave a Reply