This Python program demonstrates how you can compute Quadratic Equation using a Python program when the co-efficients are defined.
The Formula for the Quadratic Equation in Standard Form is
ax2 + bx + c = 0
a,b,c are real numbers and A is not equal to zero.
How to Compute Quadratic Equation in Python ?
# Author : Abandantcode.com # Python Program to Compute quadratic equation # import math module in python import cmath a = 2 b = 5 c = 8 # calculate the discriminant dis = (b**2) - (4*a*c) minSol = (-b-cmath.sqrt(dis))/(2*a) maxSol = (-b+cmath.sqrt(dis))/(2*a) print('{0} and {1}'.format(minSol,maxSol))
Output
(-1.25-1.5612494995995996j) and (-1.25+1.5612494995995996j)
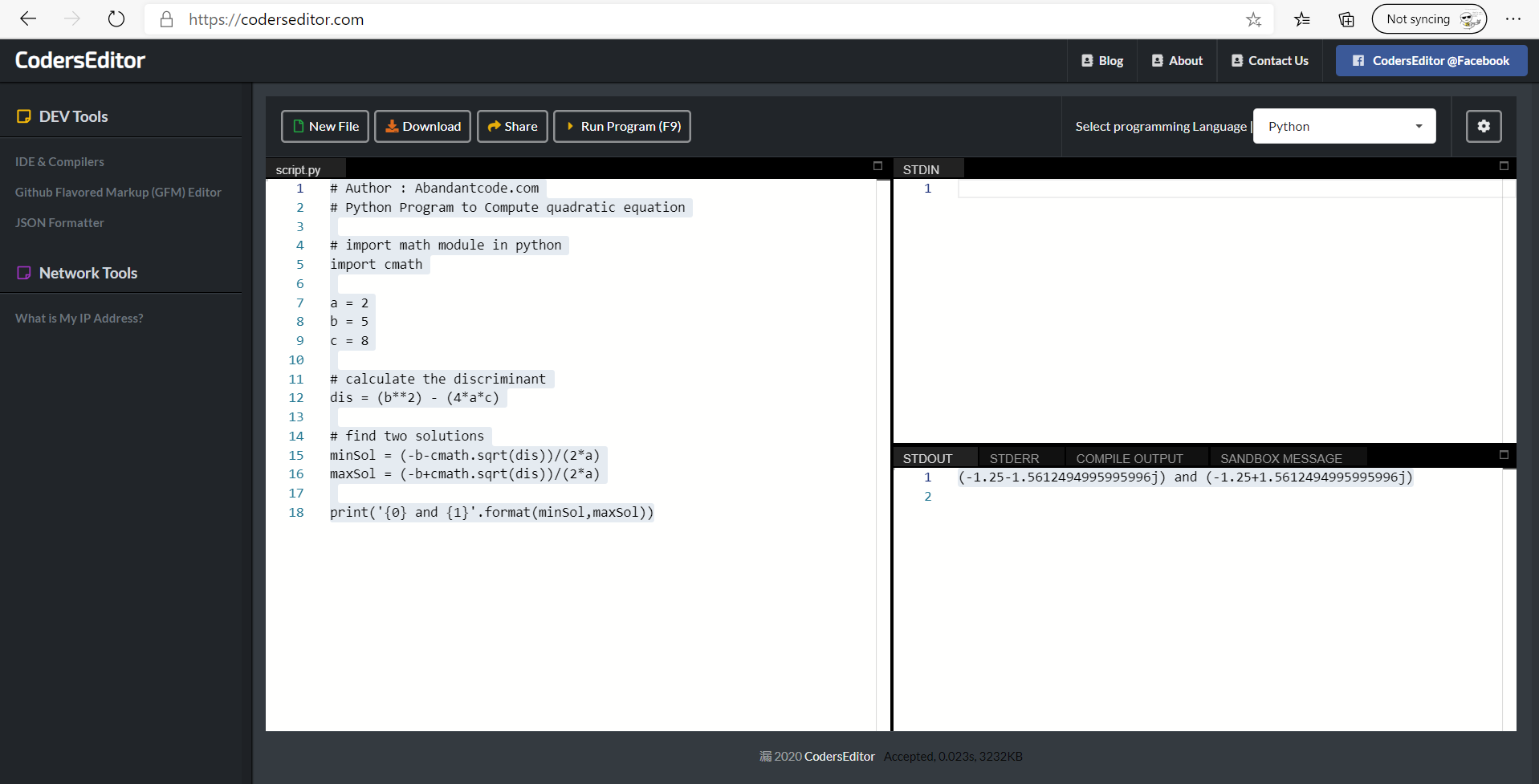
Following are the steps that we followed in the program.
- We have first imported the cmath module as we would need the complex math module to perform the square root.
- We then calculate the discriminant and then find the low and high values of the quadratic equaition.
Leave a Reply