In this Python program , you will learn how to swap two variables easily with 2 options.
- Swap two variable using a temporary variable in python.
- Swap two variable without using temporary variable in python.
How to Swap two variables in Python without using Temporary Variable?
# Author : Abundantcode.com # Python program to swap two variables without using temporary variable a = 8 b = 3 # create a temporary variable and swap the values temp = a a = b b = temp print('a : {}'.format(a)) print('b : {}'.format(b))
Output
a : 3
b : 8
How to Swap Two variables without using Temporary variable in Python?
There are multiple ways in Python to swap two variables without using a temporary variable.
Using Python Construct “,”
# Author : Abandantcode.com # Python Program to swap two variables without using temporary variables a = 5 b = 10 a, b = b, a print("a =", a) print("b =", b)
Using Arithmetic Operators
# Author : Abandantcode.com # Python Program to swap two variables without using temporary variables a = 8 b = 3 a = a + b b = a - b a = a - b print("a =", a) print("b =", b)
Swapping the numbers using the arithmetic operator works only for the numbers and you can use any arithmetic operator to achieve the same.
Output
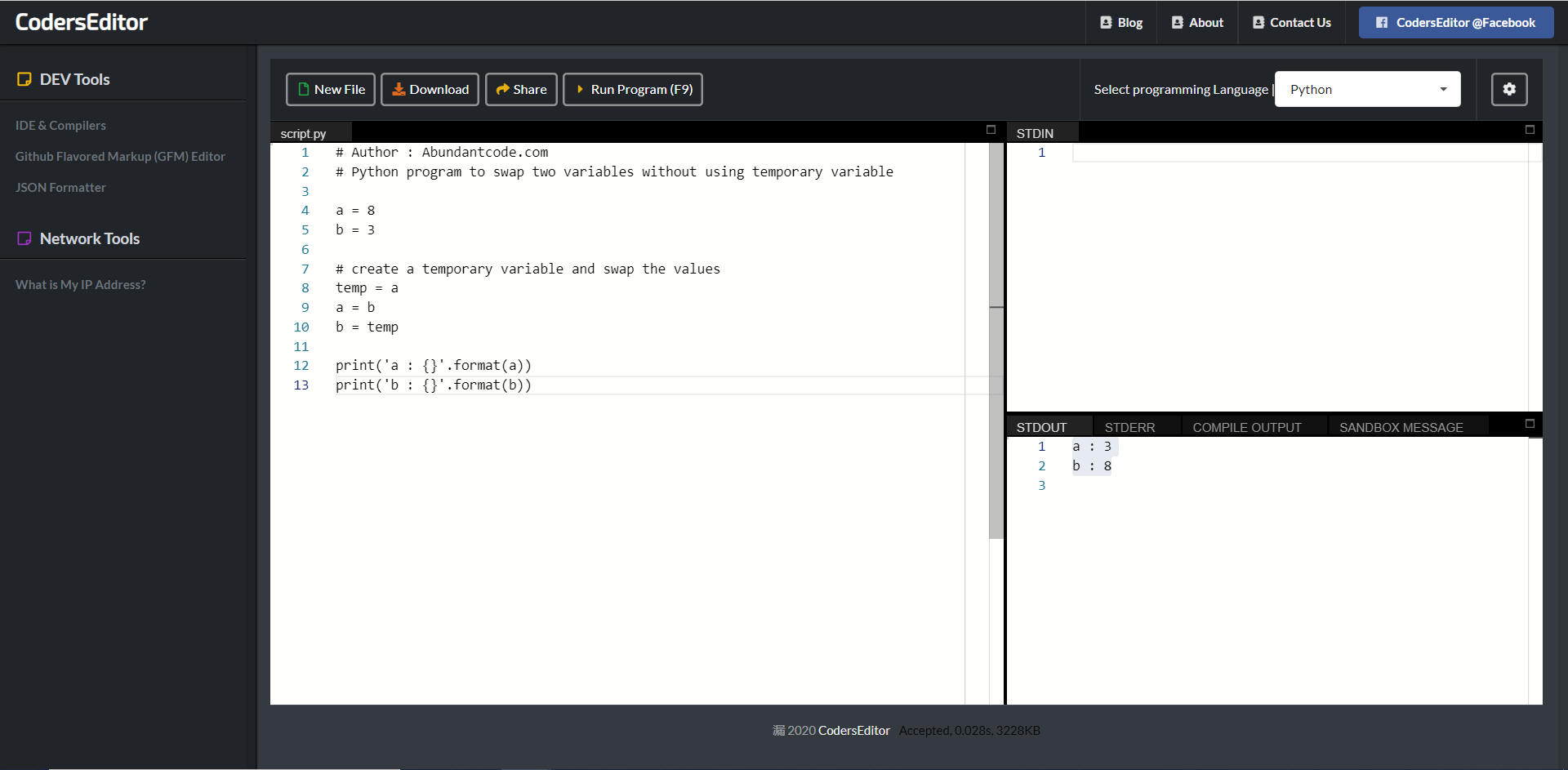
Leave a Reply