Problem
Write a program in C++ programming language to Calculate Compound Interest.
Solution
Interest on interest is the process of adding interest to the principal amount of a loan or deposit. It is the outcome of reinvesting interest rather than paying it out, so that interest is received on the principle amount plus previously collected interest in the next period.
Here’s a sample code snippet demonstrating how to calculate compound interest in C++.
#include<iostream> #include<math.h> using namespace std; int main() { float p,r,t,ci; cout<<"Enter Principle Amount:"; cin>>p; cout<<"\nEnter Rate of Interest:"; cin>>r; cout<<"\nEnter Time Period:"; cin>>t; ci = p*pow((1+r/100),t); cout<<"\nThe Calculated Compound Interest is = "<<ci<<"\n"; return 0; }
Output
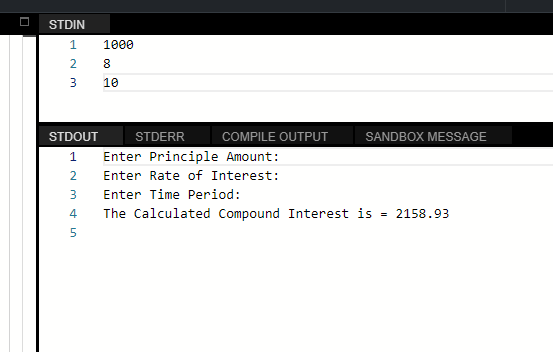
Leave a Reply