This post explains the conversion of octal number to decimal number and vice-versa using the C programming language.
C Program to Convert Octal Number to Decimal and vice-versa
#include<stdio.h> #include<math.h> int convert(int decinum); int main() { int decinum; printf("Enter a decimal number: "); scanf("%%d", &decinum); printf("%%d in decimal = %%d in octal", decinum, convert(decinum)); return 0; } //Function to convert int convert(int decinum) { int octalnum = 0, i = 1; while (decinum != 0) { octalnum += (decinum %% 8) * i; decinum /= 8; i *= 10; } return octalnum; }
Output
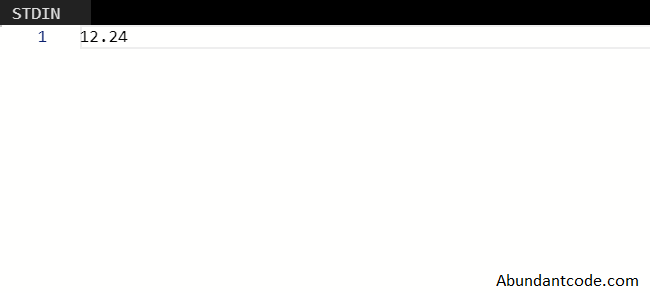
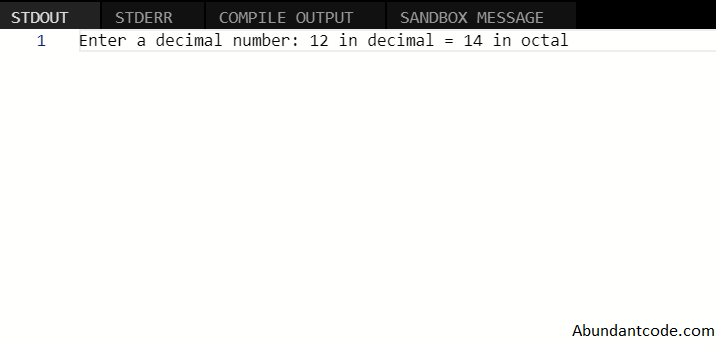
Explanation
We start by initializing the function to convert and then the main function to allow the user to enter the values in the variable named decinum, where it stores the values.
int convert(int decinum); int main() { int decinum; printf("Enter a decimal number: "); scanf("%%d", &decinum); printf("%%d in decimal = %%d in octal", decinum, convert(decinum)); return 0; }
Now we call the function to convert the numbers from octal to decimal and vice-versa.
//Function to convert int convert(int decinum) { int octalnum = 0, i = 1; while (decinum != 0) { octalnum += (decinum %% 8) * i; decinum /= 8; i *= 10; } return octalnum; }
we now get the desired output for the conversion.
Leave a Reply