Problem
Write a program in C++ to find the square root of a number in C++.
Solution
To find the square root of a number in C++, you can use the sqrt() function.
The sqrt() function defined in the math.h header file can be used to calculate the square root in C++. This function takes a number as an argument and returns its square root.
Here’s a sample code snippet showing how you can find the square root of a number in C++ using sqrt() function.
#include<iostream> #include<math.h> using namespace std; int main() { float sq,n; cout<<"Enter any positive number:"; cin>>n; sq=sqrt(n); cout<<"\nSquare root of Entered Number = "<<sq; return 0; }
Output
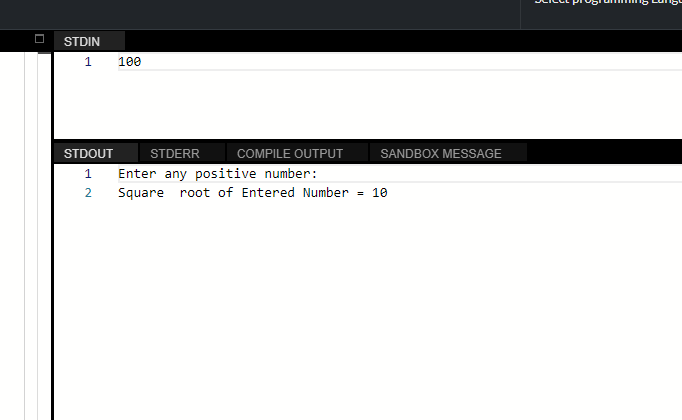
Leave a Reply