You can get the IP address of the local machine or localhost using C# using the Dns class’s GetHostByName defined in the System.Net namespace.
The Dns.GetHostByName returns the array of AddressList which contains the IP address .
How to get the IP Address of the Local Machine using C# ?
Below is the sample code snippet demonstrating the retrieval of the IP address of the local machine or localhost in C#.
using System; using System.Collections.Generic; using System.Linq; using System.Net; namespace AbundantCodeConsoleApp { internal class Program { private static void Main(string[] args) { // Get the Name of the Host string host = Dns.GetHostName(); // Get the IP address string ip = Dns.GetHostByName(host).AddressList[0].ToString(); // Display the IP Console.WriteLine("Local Host IP is " + ip); Console.ReadLine(); } } }
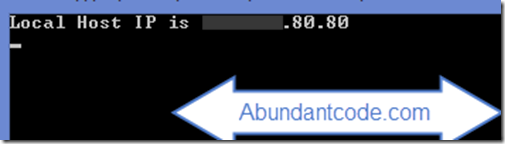
Leave a Reply