This post helps add two matrices using multi-dimensional arrays in the C programming language.
C Program to Add Two Matrices Using Multi-dimensional Arrays
#include <stdio.h> int main() { int A[10][10],B[10][10],C[10][10],i,j,row,col; printf("\n Enter row and column: "); scanf("%%d %%d",&row,&col); printf("\n Enter A matrix"); for(i=0;i<row;i++) { for(j=0;j<col;j++) { scanf("%%d",&A[i][j]); } } printf("\n Enter B matrix"); for(i=0;i<row;i++) { for(j=0;j<col;j++) { scanf("%%d",&B[i][j]); } } printf("\n Sum of the two matrices: \n "); for(i=0;i<row;i++) { for(j=0;j<col;j++) { C[i][j]=A[i][j]+B[i][j]; printf("%%d \t",C[i][j]); } printf("\n"); } return 0; }
Output
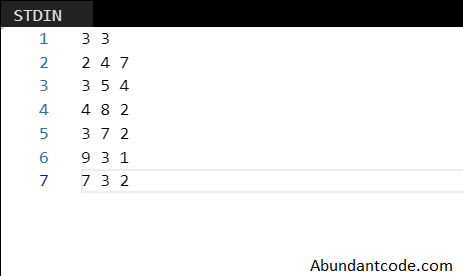
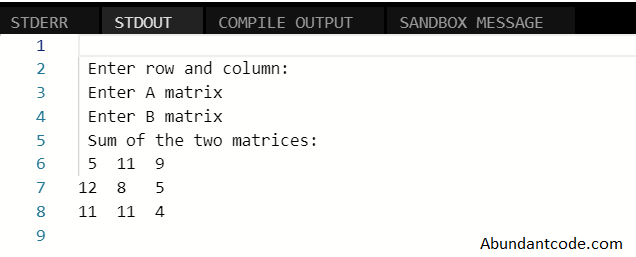
Explanation
In this C program, we have initialized several variables to do the calculation. Here, the variables initialized are A, B, C used as an array of size for the matrices and i, j, row, and col used for differentiating the rows and columns of the matrix. We also allow the user to enter the rows and columns for both the matrices.
int A[10][10],B[10][10],C[10][10],i,j,row,col; printf("\n Enter row and column: "); scanf("%%d %%d",&row,&col); printf("\n Enter A matrix"); for(i=0;i<row;i++) { for(j=0;j<col;j++) { scanf("%%d",&A[i][j]); } } printf("\n Enter B matrix"); for(i=0;i<row;i++) { for(j=0;j<col;j++) { scanf("%%d",&B[i][j]); } }
Now we proceed further for the calculation part, where we add, both the matrix A and B, the following will be displayed in the C matrix.
printf("\n Sum of the two matrices: \n "); for(i=0;i<row;i++) { for(j=0;j<col;j++) { C[i][j]=A[i][j]+B[i][j]; printf("%%d \t",C[i][j]); }
We have now obtained the fancied output.
Leave a Reply