This post helps to search a number in an array using the C programming language.
C Program to search a number in an array
#include<stdio.h> #define MAX_SIZE 100 int main() { int arr[MAX_SIZE]; int size,i,search,found; printf("\n Enter Size of an array:"); scanf("%%d",&size); printf("\n Enter elements in an array:"); for(i=0;i<size;i++) scanf("%%d",&arr[i]); printf("\n Enter element to search:\n"); scanf("%%d",&search); found=0; for(i=0;i<size;i++) { if(arr[i]==search) { found=1; break; } } if(found==1) printf("\n position found %%d",search,i+1); else printf("\n position not found %%d",search); return 0; }
Output
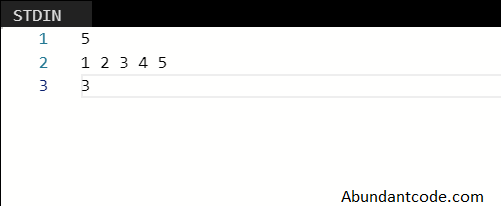
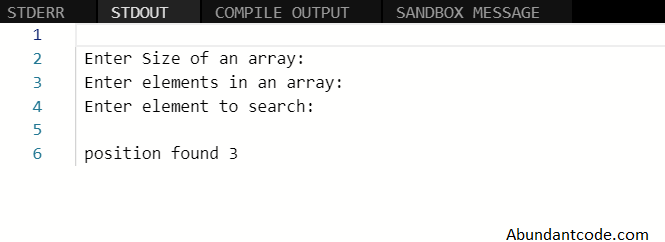
Explanation
In this program we enter the size of an array, enter an element in the array, and enter an element to search in the array. Here the variables used are arr[MAX_SIZE], size, i, search, found.
int arr[MAX_SIZE]; int size,i,search,found; printf("\n Enter Size of an array:"); scanf("%%d",&size); printf("\n Enter elements in an array:"); for(i=0;i<size;i++) scanf("%%d",&arr[i]); printf("\n Enter element to search:\n"); scanf("%%d",&search);
Here we use the for loop and if loop conditions to verify whether the given condition is satisfied.
found=0; for(i=0;i<size;i++) { if(arr[i]==search) { found=1; break; } }
Now we use the if condition to print whether the searched element is found or not.
if(found==1) printf("\n position found %%d",search,i+1); else printf("\n position not found %%d",search);
The desired output is received.
2 Comments