This post explains to find tanspose of a matrix using the C programming language.
C Program to Find Transpose of a Matrix
#include <stdio.h> int main() { int row,col,i,j,A[10][10],B[10][10]; printf("\n Enter the number of rows and columns of a A: "); scanf("%%d %%d", &row, &col); printf("\n Enter elements of the A: "); for (i=0;i<row;i++) { for (j=0;j<col;j++) { scanf("%%d", &A[i][j]); } } for (i=0;i<row;i++) { for (j=0;j<col;j++) { B[j][i]=A[i][j]; } } printf("\n Transpose of the A: \n"); for (i=0;i<col;i++) { for (j=0;j<row;j++) { printf("%%d \t", B[i][j]); printf("\n"); } } return 0; }
Output
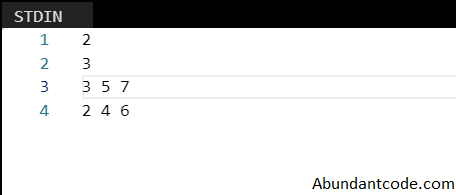
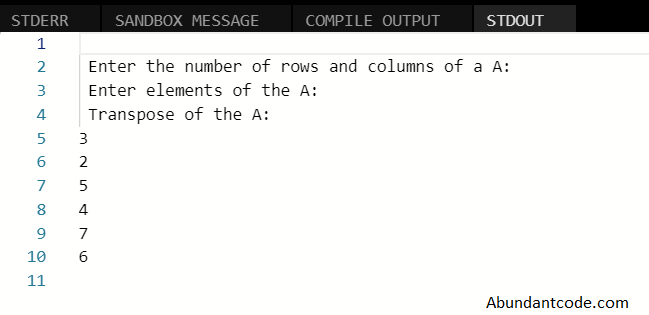
Explanation
In this C program, we transpose the elements using the following variables A, B, row, col, i, j, whereas both A and B used as the array size of the matrix, and row, col, i, j, used as the variables to differentiate the rows and columns in the matrix. We also allow the user to enter the values in the matrix.
int row,col,i,j,A[10][10],B[10][10]; printf("\n Enter the number of rows and columns of a A: "); scanf("%%d %%d", &row, &col); printf("\n Enter elements of the A: "); for (i=0;i<row;i++) { for (j=0;j<col;j++) { scanf("%%d", &A[i][j]); } } for (i=0;i<row;i++) { for (j=0;j<col;j++) { B[j][i]=A[i][j]; } }
We now proceed further with the calculation part, where we transpose the elements from columns to rows and vice-versa, and then print the terminal result.
printf("\n Transpose of the A: \n"); for (i=0;i<col;i++) { for (j=0;j<row;j++) { printf("%%d \t", B[i][j]); printf("\n"); } }
We have now obtained the fancied result/output.
Leave a Reply